Getting to know Ruby debugger
This is a guest-post by Cameron Dykes, about getting started with the ruby debugger.
A key step to debugging any program is replicating the environment to ensure you can consistently produce the bug. In my early Ruby days, to inspect the environment, I used a primitive method: placing puts
lines in my code to print values to the console (let’s call them “inspection puts”).
It may have looked something like this:
class Buggy
# assuming perform_operation and special_options exist...
def buggy_method(param=nil, options={})
puts "\n\n\nDEBUG"
puts "param: #{param}"
puts "options: #{options}"
@instance_var = perform_operation(special_options(param, options))
end
end
The case for ruby-debug
This method very easily gives me the information I need, but it has some downsides:
- To inspect the return value of
special_options
I have to add another inspection puts. - Every addition of a new
puts
requires that I restart the application to inspect the results. - To inspect how
special_options
andperform_operation
are handling the data, I have to add inspection puts inside of them. - I must remember to remove all of the inspection puts before I push the code.
If only there was a better way to do this.
ruby-debug to the rescue! By putting a breakpoint in our code, we have the ability to inspect environment state, check the return value of any methods, and step through our code one line at a time. This is much more versatile than inspection puts because the full environment is available to us. The “I wonder what the value of this is” problem is gone since we can inspect whatever we want. The step functionality the debugger gives us is useful as well, allowing us to step inside of a called method while maintaining the interactive environment.
Setting up ruby-debug
To get set up with the debugger, we’ll need to install the gem:
# Using MRI-1.9.2
gem install ruby-debug19
# Using MRI-1.8.7 or Ruby Enterprise Edition
gem install ruby-debug
ruby-debug in action
Let’s update the example from above using a debugger breakpoint instead of inspection puts:
class Buggy
# assuming perform_operation and special_options exist...
def buggy_method(param=nil, options={})
require 'ruby-debug'; debugger
@instance_var = perform_operation(special_options(param, options))
end
end
The next time the method is called, the debugger will stop and give us an interactive shell. We can inspect the values of each variable with the eval
command:
eval param
eval options
We can also see what the return value is of the invoked methods:
eval special_options(param, options)
eval perform_operation(special_options(param, options))
We can even use the step command to enter inside of special_options
and perform_operation
to see what they do.
Here are the various debugger commands that I most commonly use:
list, l
- show the code for the current breakpointeval, e
- evaluate expression and print the valuestep, s
- next line of code, moving within methodscontinue, c
- continue in the program until the program ends or reaches another breakpointquit, q
- abort the program
Many more commands are available, which can be seen by entering help
in the debugger.
Better debugging ftw!
With the ruby-debug gem, we have a better tool for diving in to our code than inspection puts. Using a debugger breakpoint, we can interactively step through our code with less cleanup.
Happy debugging!
Debugging references
- Debugging with ruby-debug in the Debugging Rails Applications guide
- ruby-debug19 gem
- Example code from this post
Who am I?
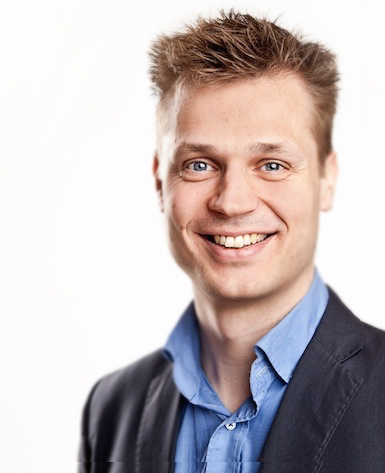
Hi, I'm Laust Rud Jacobsen, an experienced webapp developer specializing in Elixir, Ruby and PostgreSQL-based solutions. You can hire me to build awesome stuff for you. If you have any questions or comments, reach out to @object.io or privately at work@valuestream.io.