Simple git workflow with hack and ship
I use git as my primary version control tool for all internal development, configuration files, and collaborative development. As branches are virtually free with git, it makes a lot of sense to create short-lived feature-branches for each new thing you start working on. This does mean a bit of shuffling back and forth to integrate changes from others in your local work, but this “pull changes and rebase my work” workflow can be greatly eased by these small scripts.
For more than a year I’ve been using two small shell-scripts called hack
and ship
to manage local feature-branches. Hat-tip goes to ReinH for the original version of these. Notice these shortcuts are only usable when you work on a feature branch based on master, not remote branches in general.
hack
pulls down the latest changes from the central origin/master
branch, and rebases your local feature-branch on this new master
. The end result is all the latest changes are integrated, and you will be able to push your commits without adding an unnecessary merge-commit to the shared history.
#!/bin/sh -x
# Exit if any error is encountered:
set -o errexit
# git name-rev is fail
CURRENT=`git branch | grep '\*' | awk '{print $2}'`
git checkout master
git pull --rebase origin master
git checkout ${CURRENT}
git rebase master
ship
is a quick way to merge your current branch to master
, and push the result to the central repository branch called origin/master
.
#!/bin/sh -x
# Exit if any error is encountered:
set -o errexit
# git name-rev is fail
CURRENT=`git branch | grep '\*' | awk '{print $2}'`
git checkout master
git merge ${CURRENT}
git push origin master
git checkout ${CURRENT}
Usually when a feature is completed, I run hack
, run all code-tests for the project, the run ship
. Taken together, the process is automated and looks like this:
hack && rake && ship
where rake
runs all relevant tests, and exits with a non-zero error-code. If one or more tests fail, the changes are not shipped (due to the nature of &&
between the commands), and a fix can be committed before sharing the changes with other developers.
What is your process for managing feature branches in git?
Update: See also the chop script.
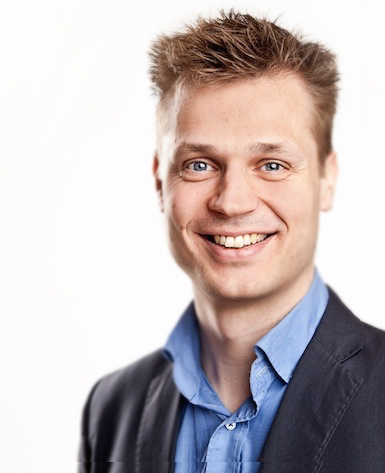
Hi, I'm Laust Rud Jacobsen, an experienced webapp developer specializing in Elixir, Ruby and PostgreSQL-based solutions. You can hire me to build awesome stuff for you. If you have any questions or comments, reach out to @object.io or privately at work@valuestream.io.